便签列表活动
职责
组件
- public static final int ADD = 1; 添加请求编码
- public static final int EDIT = 2;修改请求编码
- RecyclerView recyclerView; 列表
- LinearLayoutManager linearLayoutManager; 列表布局
- NodeAdapter myAdapter;列表适配器
- ArrayList datas;当前便签集合
- NoteDAO noteDAO;数据层
事件
- 需要实现项容器RelativeLayout单击事件
- 需要实现项容器RelativeLayout长按事件
MainActivity的onCreate时序图
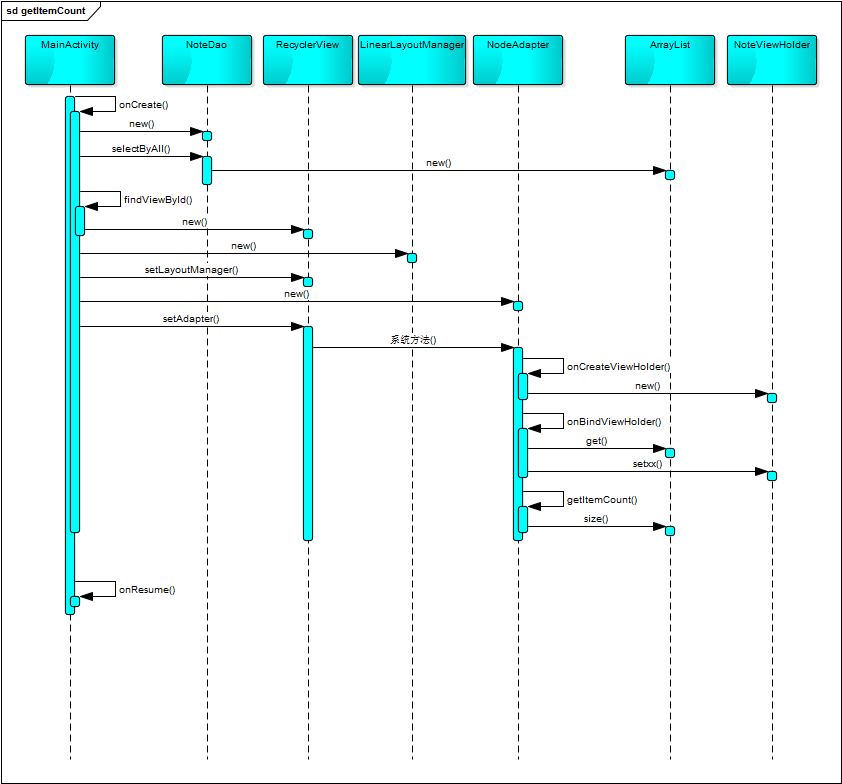
res/layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
tools:context=".MainActivity">
<android.support.design.widget.AppBarLayout
android:layout_height="wrap_content"
android:layout_width="match_parent"
android:theme="@style/AppTheme.AppBarOverlay">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
android:theme="@style/AppTheme.AppBarOverlay"
app:popupTheme="@style/AppTheme.PopupOverlay"/>
</android.support.design.widget.AppBarLayout>
<include layout="@layout/content_main"/>
</android.support.design.widget.CoordinatorLayout>
res/layout/content_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
app:layout_behavior="@string/appbar_scrolling_view_behavior"
tools:showIn="@layout/activity_main"
tools:context=".MainActivity">
<android.support.v7.widget.RecyclerView
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
</android.support.v7.widget.RecyclerView>
</LinearLayout>
res/menu/menu_main.xml
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
tools:context=".MainActivity">
<item android:id="@+id/add"
android:title="@string/add"
android:orderInCategory="100"
android:icon="@drawable/ic_add"
app:showAsAction="always"/>
</menu>
MainActivity.java
package com.hzj163.mysqlitedb;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.support.v4.app.DialogFragment;
import android.support.v7.app.AlertDialog;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.support.v7.widget.Toolbar;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Toast;
import com.hzj163.mysqlitedb.adapter.NodeAdapter;
import com.hzj163.mysqlitedb.beans.Note;
import com.hzj163.mysqlitedb.db.NoteDAO;
import com.hzj163.mysqlitedb.viewholder.NoteViewHolder;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity implements NoteViewHolder.ItemonClick, NoteViewHolder.ItemonLongClick {
//添加的code
public static final int ADD = 1;
//修改的code
public static final int EDIT = 2;
RecyclerView recyclerView;
LinearLayoutManager linearLayoutManager;
NodeAdapter myAdapter;
ArrayList<Note> datas;
NoteDAO noteDAO;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//实例化Toolbar
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
//设置Toolbar属性
toolbar.setTitle("小便签");
//激活Toolbar
setSupportActionBar(toolbar);
//实例化noteDAO
noteDAO = new NoteDAO(this);
//初始化数据集合
datas = noteDAO.selectByAll();
//初始化recyclerView
recyclerView = (RecyclerView) findViewById(R.id.recyclerView);
//初始化linearLayoutManager
linearLayoutManager = new LinearLayoutManager(this);
//设置recyclerView布局
recyclerView.setLayoutManager(linearLayoutManager);
//初始化适配器
myAdapter = new NodeAdapter(datas, this, this);
//设置recyclerView适配器
recyclerView.setAdapter(myAdapter);
}
//菜单创建
@Override
public boolean onCreateOptionsMenu(Menu menu) {
//设置菜单【让xml文件变成menu对象】
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
//菜单事件【添加便签】
@Override
public boolean onOptionsItemSelected(MenuItem item) {
//获得当前点击的菜单的ID
int id = item.getItemId();
//添加便签事件
if (id == R.id.add) {
//跳转到一个活动
Intent intent = new Intent(this, AddActivity.class);
//具备返回值的跳转
startActivityForResult(intent, ADD);
return true;
}
return super.onOptionsItemSelected(item);
}
//活动返回
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
//处理添加便签
if (requestCode == ADD && resultCode == ADD) {
//需要Note支持序列化
Note temp = (Note) data.getSerializableExtra("note");
//判断内容是否不为空
if (temp.getInfo().trim().equals("")) {
Toast.makeText(this, "便签内容不可以为空", Toast.LENGTH_LONG).show();
return;
}
//数据库添加
noteDAO.insert(temp);
//集合添加【需要在最上面一行插入】
datas.add(0, temp);
//更新
myAdapter.notifyDataSetChanged();
}
//处理修改便签
if (requestCode == EDIT && resultCode == EDIT) {
Note temp = (Note) data.getSerializableExtra("note");
if (temp.getInfo().trim().equals("")) {
Toast.makeText(this, "便签内容不可以为空", Toast.LENGTH_LONG).show();
return;
}
noteDAO.update(temp);
//这里需要Note对象的equals方法给出相等规则
//datas.indexOf(temp)按照对象查询出所在集合的下标,依据的标准是对象的equals方法
int position = datas.indexOf(temp);
//修改集合
datas.set(position, temp);
//更新【采取局部更新notifyItemChanged】
myAdapter.notifyItemChanged(position);
}
}
//每一项的点击事件
@Override
public void onClick(View v, int position) {
//修改便签
Intent intent = new Intent(this, EditActicity.class);
//携带当前选中的便签对象,传递给修改界面
intent.putExtra("note", datas.get(position));
startActivityForResult(intent, EDIT);
}
//每一项的长按事件
@Override
public void onLongClick(View v, int position) {
//删除便签
final int tempPosition = position;
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("删除确认");
builder.setMessage("你确实要删除吗?");
builder.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
//数据库删除
noteDAO.del(datas.get(tempPosition).getId());
//集合维护
datas.remove(tempPosition);
//更新【采取局部删除notifyItemRemoved】
myAdapter.notifyItemRemoved(tempPosition);
}
});
builder.setNegativeButton("取消", null);
AlertDialog alertDialog = builder.create();
alertDialog.show();
}
}